Angular framework for frontend development
Angular: is a framework for front end development. We can write plain vanilla javascript code and develop our application but as the application complexity increases, it is hard to maintain the large chunk of javascript code. Moreover the application developed using plain vanilla javascript is hard to test. Angular gives our application a clean and loosely coupled structure that is easy to understand and easy to maintain. Apart from this it also gives us lots of utility code which we can reuse in a lot of applications specifically related to navigation and maintaining browser history.
The area where angular excels is its ability to create single page applications (SPA). In a single page application, there is only one page and the components and layout of that page keep changing at runtime as per the user's actions, unlike multi page applications where there are multiple pages and altogether a new page gets rendered from the server every time a user performs some action.

Understand the single page application concept:
In single page applications like we make in Angular, there is only a single page and this page is divided into multiple components. Now when a user performs any action, only a relevant component of the page gets updated and the rest of the page remains the same, unlike a multi page application where the entire page gets served by the server upon user action.
If you see the project structure of an angular app, there is a global page (index.html and style.css) and a default app component and app module. Later you can create as many modules and components under the root module of the project. In the angular project structure, there is only a single style.css and index.html which are the global ones. Later you can create multiple modules and components under the root module. So during user actions, the main page remains the same, only the particular component gets changed belonging to a particular app module.
Anything written in style.css applies to the entire page, while anything written in appcomonent.css applies to a particular component. There can be multiple app modules and each app module can have hundreds of components.
A module in angular is a container for a group of related components. In other words a module is a collection of related components.
Every component has its own HTML, CSS, and TS (transcript) files.
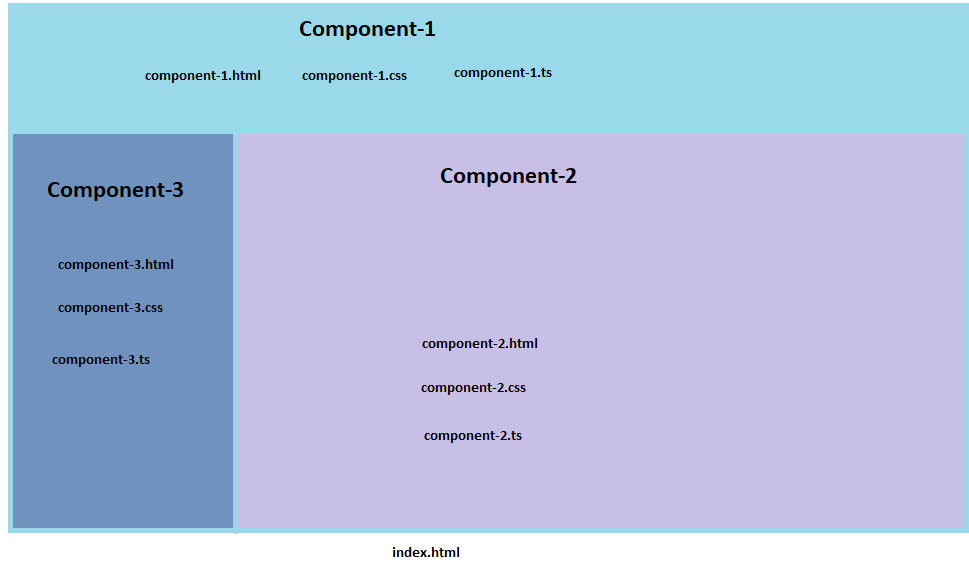
Getting started with Angular:
1. First install NodeJS. Node JS provides us a tool called NPM (Node Package Manager) with the help of which we can install various third party libraries for developing angular applications. One such library is Angular CLI (Command Line Interface). It is a command line tool which we use to create a new angular project. Angular CLI also includes a server which we can run on our local host.
2. Use below command in command prompt or terminal to install Angular CLI.

3. Now create a new angular project with the help of Angular CLI command:
Open the terminal and type below CLI command:
ng new projectname
This command will install necessary angular npm packages and dependencies and create a new default angular application which can be launched at http://localhost:4200/
The default project structure can be seen in the image below under the section - 'Understand the angular project structure'.
4. To launch this application, go to the location where the workspace is created using
cd location
Here 'location' is the path where project workspace is residing.
and then type below CLI command:
ng serve --open
This command will launch the default application on the browser executing at http://localhost:4200/
5. Also install any editor of your choice either sublime text or visual studio etc. and open the project.
Understand the angular project structure:
Once you are done with setting up and creating the Angular project, you will see the project structure as shown below: By default there will be one app component and one module under the src folder. Later on you can create as many components and modules as needed, and register the components with a particular module.
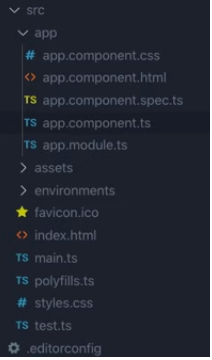
Inside the src folder, you will find one index.html which is our main page forming the single page app. This is the page that keeps on getting updated at run time as the components on this page are getting updated as per the user actions.
Style.css: This style is applicable to index.html.
Inside src folder, you will also find a default app component and an app module. This app component has its own html, css, transcript files. You can add as many components under the src and every component has its own html, css, and transcript files.
A module in angular is a container for a group of related components. In other words a module is a collection of related components.
Asset: In asset directory, you can keep all your images.
app directory: app is a default module or you can also call it as root module. You can create multiple modules under this root module.
Create Additional components using Angular CLI
Go to the terminal and reach the workspace location path with the help of cd command. Now type the below command:
ng g c TestComponent
Here the 'TestComponent' is the name of the component which can be anything as per your choice. This command will create a new component ('TestComponent' in this case) along with its html, css, transcript files under src folder. This command also register the newly created component with the app module. If you see the project structure now, you'll find that a new folder gets created under app directory.
Create additional modules in angular
Go to the terminal and reach the workspace location path with the help of cd command. Now type the below command:
ng generate module TestModule
Here 'TestModule' is the name of the module which can be anything as per your choice. In the above section we've created a component which gets created under app module, but if you want to create components under this newly created module then you can use below command:
ng generate component TestModule/newComponent
This command will generate a new component called 'newComponent' under TestModule. This way you can generate multiple components under a module.