How to find XPath in selenium?
XPath is the XML path of a web element in the DOM. Three components are needed to find an XPath of an element over a web page-
- HTML tag
- Tag attribute
- Tag value
You can inspect these values in the DOM by inspecting the element. Just right click on the element of the webpage and select inspect element.
The HTML source page will open in a separate window where you can find the tag, attributes, and its value.
Once these 3 values are known, then press Ctrl+F and write the XPath using the below format in the search field-
//tag[@attribute name='attribute value']
If the XPath is correct, then the desired element will be highlighted, and you can use this as a locator in your
automation test.
Some common HTML tags are:
div=division
span=span
p=paragraph
a=anchor tag
tr=table row
td=table data
ul=unordered list
li=list item
ol=ordered list
h=heading
label
input
and so on.
And some of the common attributes are class, id, name, href, title, etc.
For e.g. a div tag has a 'class' attribute, or 'id' attribute, etc.
An anchor tag 'a' must have an 'href' attribute. These attributes must have value. A value is nothing but a name given to the attribute so that it can be identified in the DOM.
Suppose there is a division in html whose class attribute value is "abcd", so we'll write like this:
//div[@class="abcd"]
It means, finds a division tag in the HTML whose class attribute value is "abcd".
If we write like this //*[@class="abcd"] i.e. putting an asterisk in place of the tag, this means,
find any tag in the HTML DOM whose class attribute value is "abcd".
If we write with a dot operator like .//*[@class="abcd"] here the dot represents the context node. It means the processing starts from the current node. To be more precise find any tag in the HTML whose class attribute value is "abcd" and start processing from the current node.
If we do not use dot and simply write //*[@class="abcd"] then it will search class with value "abcd" in the entire document.
If you further want to go inside a parent tag, then you can use a single slash in the middle of the XPath
//div[@class="abcd"]/ul/li/a this means under the parent division whose class value is "abcd" find an anchor tag which is under ul and li tags.
All these xpaths represent either an element or a list of elements on the web page.
What are XPath axes?
There are times when deriving the XPath is not straightforward, this may be due to the dynamic attributes and values of the HTML tag or complex
and nested structure of the DOM elements with common names given to the attribute values. To counter such challenges, we use XPath axes.
An XPath axis is a one-word statement that represents the relationship between the current node and its relative node.
With the help of an XPath axis, we can find the relative nodes of a current node.
Following are the common XPath axes, that represents the relationship between the current node and its relative node:
1. following
2. following-sibling
3. child
4. descendant
5. ancestor
6. preceding
7. parent
If the attribute value is dynamic, then we can make use of below XPath functions:
1. contains()
2. starts-with()
3. text()
What is contains() method in XPath?
The contains() is an xpath function that fetches the tags containing some particular pattern. Suppose there is an attribute value 'btn123', and the numeric figure keeps changing, and is not constant.
So you can write
.//*[contains(@name, 'btn')]
This means find any tag starting from the current node whose name contains 'btn'.
You can also use, OR and AND operator inside your XPath to put a conditional check.
For e.g.
//*[@type='submit' or @name='abcd'],
This means select any tag whose type is 'submit' or name is 'abcd'.
//*[@type='submit' and @name='abcd'],
This means select any tag whose type is submit and name is 'abcd'. The satisfaction of both conditions is necessary.
What is starts-with() method in XPath?
The starts-with() is also an XPath function, that fetches the tags whose attribute value starts with a specific pattern. For e.g.
//label[starts-with(@id,'abcd')]
It means to find a label whose id starts with 'abcd'.
What is text() method in XPath?
The text() is an XPath function that fetches the HTML tag whose underlying text exactly matches with the one specified in the XPath statement. The below statement says- Find a table data whose text= 'abcd'.
.//td[text()='abcd']
What is the following axis in XPath?
The following xpath axis means find all the elements in the DOM starting after a particular node For. e.g.
//*[@class='post-content']//following::a This means to find all the anchor tags after 'post-content' class.
You can see, it is giving 18 anchor tags after 'post-content' class.
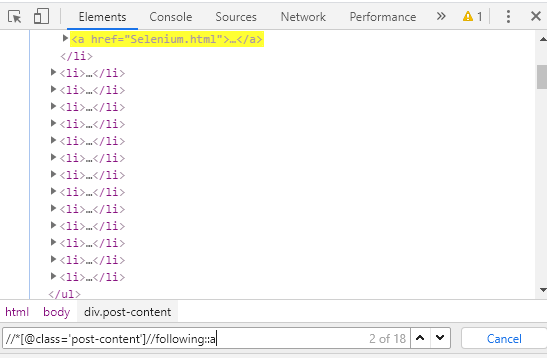
But what if you want an anchor tag present at a particular position? For this, you can specify the index as below.
//*[@class='post-content']//following::a[9]
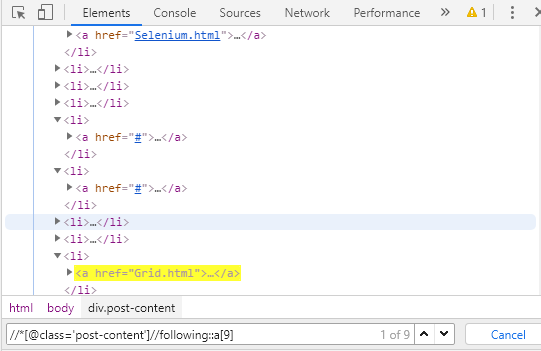
What is an ancestor in XPath?
An ancestor axis in the XPath will find parent as well as grand parent nodes of the current tag. For e.g.
//*[@class='logoCotainer']//ancestor::div
This means find all the parent as well as grand parent division tags of any tag whose class attribute has value ='logoCotainer'.
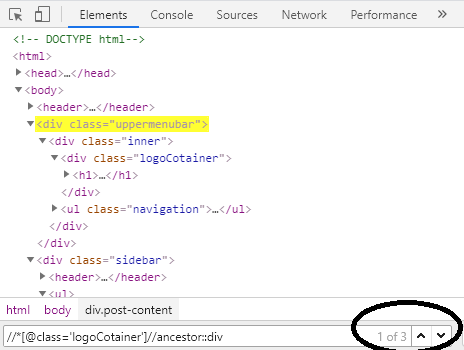
What is a descendant in XPath?
A descendant axis will find all children as well as grandchildren elements of the current node. A child element is the one that is nested inside its parent tag. Whereas if a tag is further nested inside the child tag, then this will be called a grandchild. For e.g.
//*[@class='uppermenubar']//descendant::div
This means to find all the child divisions as well as grandchild divisions of the class 'uppermenubar'.
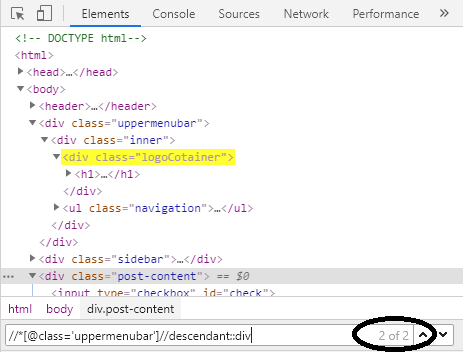
What is preceding in XPath?
A preceding axis in the XPath will find all the elements in the DOM before a particular node except its ancestors. For. e.g.
//*[@class='navigation']//preceding::div
This means find all the divisions of any tag whose class attribute has value as 'navigation' except its ancestors. Here, since 'inner' and 'uppermenubar' are the ancestors for 'navigation' class therefore they are excluded.
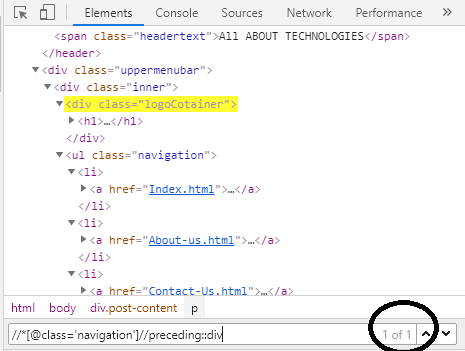
What is a child in XPath?
A child axis will find all the child nodes of its parent tag. The child nodes are the ones that are nested inside the parent tag. for e.g
//*[@class='uppermenubar']//child::div
This means to find all child divisions (div) of class 'uppermenubar'
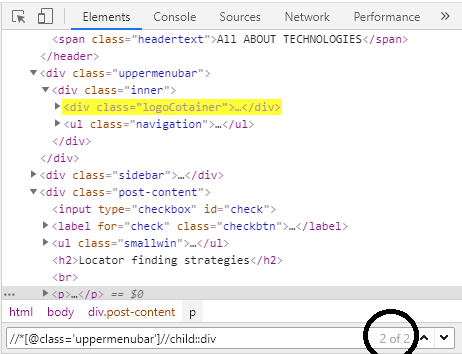
What is a parent in XPath?
The parent axis in XPath will find the parent node of the current tag. For e.g.
//*[@class='navigation']//parent::div
This means find the parent division of any tag whose class attribute has value as 'navigation'.
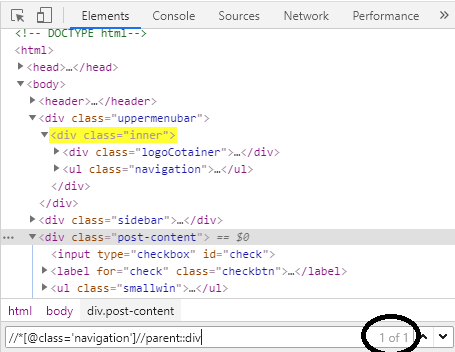