Scope Rules in Java | What are scope rules for class members and local variables in Java?
In java, every class has members in terms of variables, constants, methods, and objects. To access these members from within the class, OR outside the class, java provides explicit accessibility modifiers to control the accessibility of members in a class.
Below are the accessibility modifiers provided by Java -
public
protected
default
private
The access to public, protected, default, and private members are governed by specific scope rules. Two such scope rules are -
-Class scope for members, and
-Block scope for local variables
What is the Class scope for members in Java?
The class scope for members defines the rules as to
how member declarations are accessed within the class. It defines how to access the members including the inherited ones from code within a
class.
Static members belong to a class and not to any object of the class therefore static code cannot be
executed in the context of an object, therefore 'this' and 'super' references are not available for static members.
Also, a static block cannot access the non-static (instance) members by their simple names but on the contrary
static members can be accessed within a non-static block because the object has the knowledge of its class.
1. Static variable can be accessed by instance method of a class
2. Instance variables cannot be accessed by their simple names within a static method of a class
3. Static method can be called/accessed within an instance method of a class
4. Instance method cannot be called or accessed by their simple names within a static method of a class
5. 'this' reference keyword cannot be used in a static context/block/static method.
6. 'super' reference keyword cannot be used in a static context/block/static method.
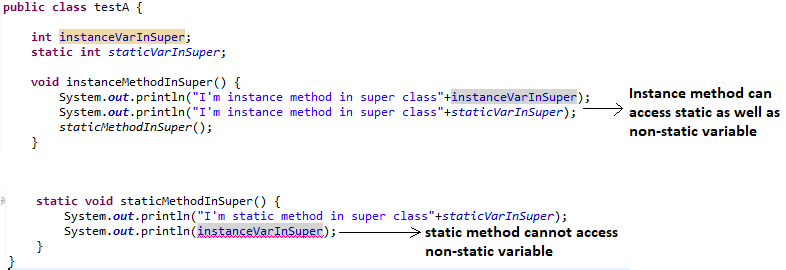
What is the block scope for local variables in Java?
Block scope for local variables defines the scope as to how local variable declarations are accessed within a block.
In java, the grouping of 'declarations' and 'statements' inside braces {} forms a block. Blocks can be nested and certain
scope rules are applicable to local variables declared in such blocks.
1. A local variable can be declared
anywhere inside a block.
2. A local variable declared inside a block is in scope or accessible within the block
in which it is declared but it is not accessible outside of this block.
3. In a method, the parameters declared in the method parenthesis () along with the variables declared inside
method block {} comprised of local variables.
These local variables cannot be redeclared again in the same block.
4. The method parameters cannot be redeclared in the method body.
5. In the case of nested blocks, the variables declared in the outer block are visible in the inner block but the variables declared in
the inner block cannot be accessible in the enclosing or outer block.
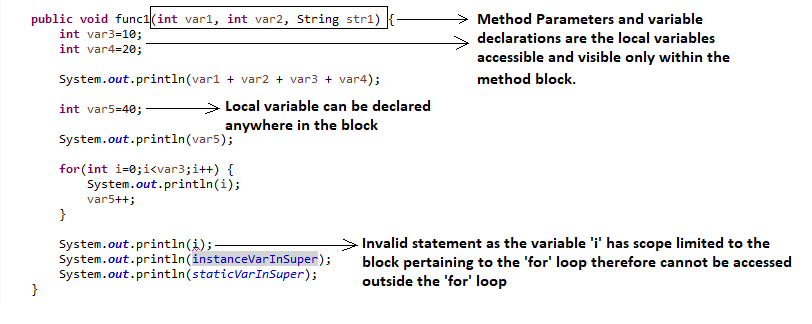