Constructor in Java
The core function of the constructor is to set the initial state of an object when the object is created by using the new operator. Constructor has the same name as that of the class. It doesn't return anything and thus no return type is allowed for the constructor (not even void). The constructor may have an explicit accessibility modifier. And a constructor can take parameters. The syntax of a constructor is as shown below:
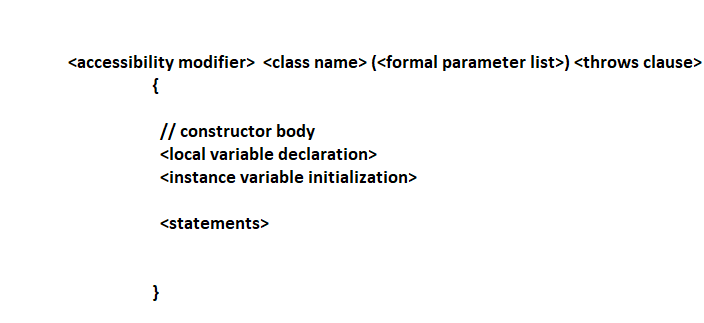
Default constructor in Java
If there is no explicit constructor specified in a class then an implicit default constructor is supplied for the class. The default constructor doesn't have any parameters and the only purpose of the default constructor is to call the superclass constructor. This is to ensure that the inherited state of the object is initialized properly. Moreover, the default constructor initializes the instance variables in the object with the default values of their type. If a class has an explicit constructor specified in the class body then the default constructor is no longer needed to set the initial state of the object.
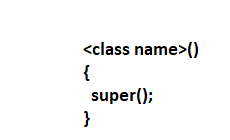
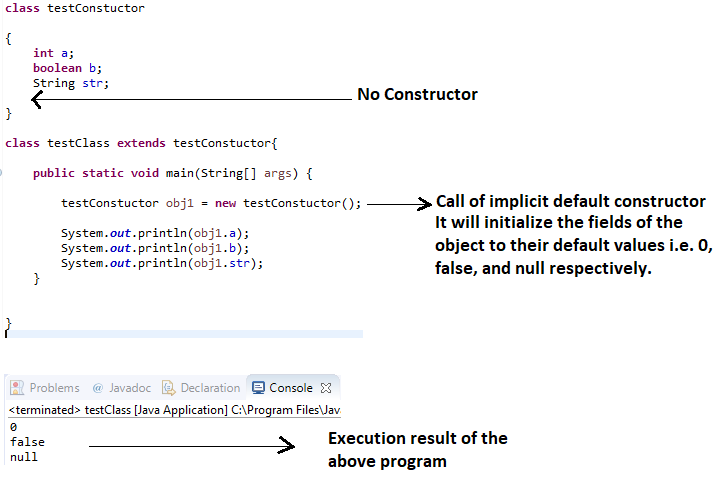
Constructor Overloading in Java
Constructors in java can also be overloaded like methods. Since constructors in a class have the same name as the class, their signatures are differentiated by their parameter list. Constructors cannot be inherited or overridden. They can be overloaded but only in the same class. Since constructors have the same name as that of the class, therefore each parameter list must be different when defining more than one constructor for a class.
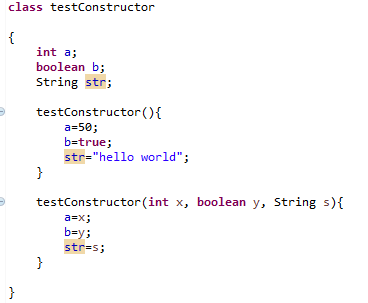
Constructor Chaining in Java
One constructor invoking the other within the same class in an overloaded constructor scenario is called local constructor chaining. This can be done using the this() construct. The below code snippet illustrates the local chaining of the overloaded constructors using this() construct. Here the first constructor invokes the second which in turn invokes the third by using the this() construct. Thus the execution of the third constructor completed first, followed by the second, and lastly the execution of the first constructor that was called first.
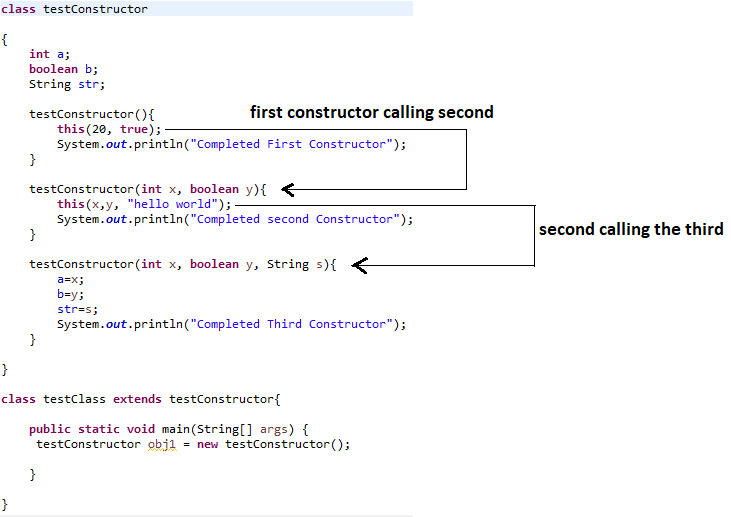
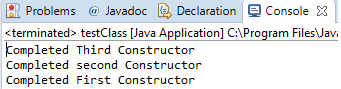
Another scenario of constructor chaining happens when the sub-class constructor invoking its immediate superclass constructor
in an inherited scenario.
The super() construct is used in a sub-class constructor to invoke a constructor in the immediate superclass.
This way, a subclass can influence the initialization of its inherited state when an object of the subclass is created.
A super() call in the constructor of a subclass invokes the relevant constructor from the superclass,
based on the signature of the call.
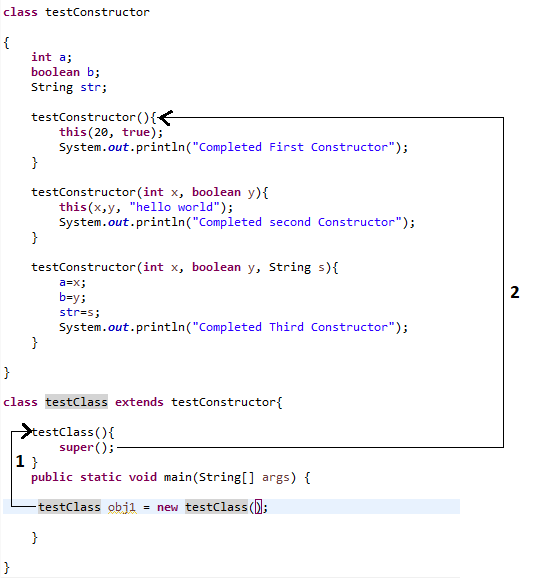