Free online test on Data structure | Self-evaluation test on Data structure
Data structure is an important topic for a person having coding aspirations. Data structure algorithms and programs are often asked in a technical interview.
This article comprises multiple choice questions and answers on Data structure. This self-evaluation test has 10 multiple-choice questions on Data structure, intended to prepare a person for technical interviews and the campus selection process.
MCQ questions and answers on Data structure
Topic- Data structure
The test consists of 10 questions.
No negative marking for this test.
No Time limit
The pass percentage is 70%
The correct answer with a description will be displayed after the answer has been marked.
Submit the test to calculate your score once you are done with all the questions.
Complexity Level- Moderate
Q1:Analyze the below program and identify the type of sorting:
public class abc {
public void sort(int arr[]) {
int n = arr.length;
for (int i = n / 2 - 1; i >= 0; i--) {
heapify(arr, n, i);
}
for (int i = n - 1; i >= 0; i--) {
int temp = arr[0];
arr[0] = arr[i];
arr[i] = temp;
heapify(arr, i, 0);
}
}
void heapify(int arr[], int n, int i) {
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && arr[l] > arr[largest])
largest = l;
if (r < n && arr[r] > arr[largest])
largest = r;
if (largest != i) {
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
heapify(arr, n, largest);
}
}
static void printArray(int arr[]) {
int n = arr.length;
for (int i = 0; i < n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
public static void main(String args[]) {
int arr[] = { 23, 1, 12, 9, 5, 6, 10, 7 };
abc hs = new abc();
hs.sort(arr);
System.out.println("Sorted array is");
printArray(arr);
}
}
public class abc {
public void sort(int arr[]) {
int n = arr.length;
for (int i = n / 2 - 1; i >= 0; i--) {
heapify(arr, n, i);
}
for (int i = n - 1; i >= 0; i--) {
int temp = arr[0];
arr[0] = arr[i];
arr[i] = temp;
heapify(arr, i, 0);
}
}
void heapify(int arr[], int n, int i) {
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && arr[l] > arr[largest])
largest = l;
if (r < n && arr[r] > arr[largest])
largest = r;
if (largest != i) {
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
heapify(arr, n, largest);
}
}
static void printArray(int arr[]) {
int n = arr.length;
for (int i = 0; i < n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
public static void main(String args[]) {
int arr[] = { 23, 1, 12, 9, 5, 6, 10, 7 };
abc hs = new abc();
hs.sort(arr);
System.out.println("Sorted array is");
printArray(arr);
}
}
Q2: Which data structure is implemented in the below program?
public class dataStructure {
int SIZE = 5;
int items[] = new int[SIZE];
int front, rear;
dataStructure() {
front = -1;
rear = -1;
}
boolean isFull() {
if (front == 0 && rear == SIZE - 1) {
return true;
}
return false;
}
boolean isEmpty() {
if (front == -1)
return true;
else
return false;
}
void addEle(int element) {
if (isFull()) {
System.out.println("DS is full");
} else {
if (front == -1)
front = 0;
rear++;
items[rear] = element;
System.out.println("Inserted " + element);
}
}
int removeEle() {
int element;
if (isEmpty()) {
System.out.println("DS is empty");
return (-1);
} else {
element = items[front];
if (front >= rear) {
front = -1;
rear = -1;
}
else {
front++;
}
System.out.println("Deleted -> " + element);
return (element);
}
}
void display() {
int i;
if (isEmpty()) {
System.out.println("Empty DS");
} else {
System.out.println("\nFront index-> " + front);
System.out.println("Items -> ");
for (i = front; i <= rear; i++)
System.out.print(items[i] + " ");
System.out.println("\nRear index-> " + rear);
}
}
public static void main(String[] args) {
dataStructure ds = new dataStructure();
ds.removeEle();
ds.addEle(5);
ds.addEle(6);
ds.addEle(7);
ds.addEle(8);
ds.addEle(9);
ds.addEle(10);
ds.display();
ds.removeEle();
ds.display();
}
}
public class dataStructure {
int SIZE = 5;
int items[] = new int[SIZE];
int front, rear;
dataStructure() {
front = -1;
rear = -1;
}
boolean isFull() {
if (front == 0 && rear == SIZE - 1) {
return true;
}
return false;
}
boolean isEmpty() {
if (front == -1)
return true;
else
return false;
}
void addEle(int element) {
if (isFull()) {
System.out.println("DS is full");
} else {
if (front == -1)
front = 0;
rear++;
items[rear] = element;
System.out.println("Inserted " + element);
}
}
int removeEle() {
int element;
if (isEmpty()) {
System.out.println("DS is empty");
return (-1);
} else {
element = items[front];
if (front >= rear) {
front = -1;
rear = -1;
}
else {
front++;
}
System.out.println("Deleted -> " + element);
return (element);
}
}
void display() {
int i;
if (isEmpty()) {
System.out.println("Empty DS");
} else {
System.out.println("\nFront index-> " + front);
System.out.println("Items -> ");
for (i = front; i <= rear; i++)
System.out.print(items[i] + " ");
System.out.println("\nRear index-> " + rear);
}
}
public static void main(String[] args) {
dataStructure ds = new dataStructure();
ds.removeEle();
ds.addEle(5);
ds.addEle(6);
ds.addEle(7);
ds.addEle(8);
ds.addEle(9);
ds.addEle(10);
ds.display();
ds.removeEle();
ds.display();
}
}
Select the correct answer:
Q3: Which of the following statements is true for the binary search?
Q4: A data structure in which the insertion and removal of elements can be performed from both ends i.e. rear and front is ________
Q5: In a max heap, the value of a node is always ______
Q6: Which of the following statement is true for a Heap data structure?
Q7: In a doubly linked list, the transversal is possible in only one direction
Q8: Observe the below image and identify the type of Heap.
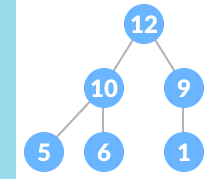
Q9: Which of the following statements is true about Heapify?
Q 10: Which of the statement is correct for a B-tree?