- Selenium
- JMeter
- DevOps
- VERSION CTRL
- ONLINE QUIZ
- Number System MCQ - 1
- Number System MCQ - 2
- Number System MCQ - 3
- Simplification MCQ - 1
- Average MCQ - 1
- Simple Interest MCQ - 1
- Compound Interest - MCQ
- Percentage - MCQ
- Ratio and Proportion - MCQ
- Profit and Loss - MCQ
- Time, Speed, and Distance - MCQ
- Time, and Work - MCQ
- Boats and Streams - MCQ
- General Knowledge Quiz - Indian Polity
- General Knowledge Quiz - History
- General Knowledge Quiz - Geography
- GK Quiz - Indian Polity - 2
- GK Quiz - Banking and Finance
- GK Quiz - Economics
- GK Quiz- Merger and Acquisition
- GK Quiz- International Bodies
- English Grammar quiz-1
- English Grammar quiz-2
- S/W PROJECT MANAGEMENT
- PROTRACTOR
- Java -Tutorials
- MORE
How to test microservices
This article discusses simplified ways to test microservices architecture-based applications. As we know that in a microservice architecture, the entire application is divided into smaller loosely coupled components called microservices. Each microservice acts as a small individual application, each having its own DB.
These microservices communicate with each other through APIs.
Advantages of having microservice-based architecture:
2. If one microservice is down, then it doesn't halt the entire system or application.
3. Deployment is relatively easy.
To test microservices architecture-based applications, we need to consider the below testing phases:
Unit testing: The developers must ensure that every piece of code component works as expected. Unit testing is the first step to check the accuracy of the individual modules.
Individual component testing: In this phase, we validate the individual component is working fine in isolation. From a testing point of view, ensure that every endpoint is touched upon. Prepare the UI test scenarios that cover every individual service working in an underlying component. In this, you are validating the request and response of a component in isolation.
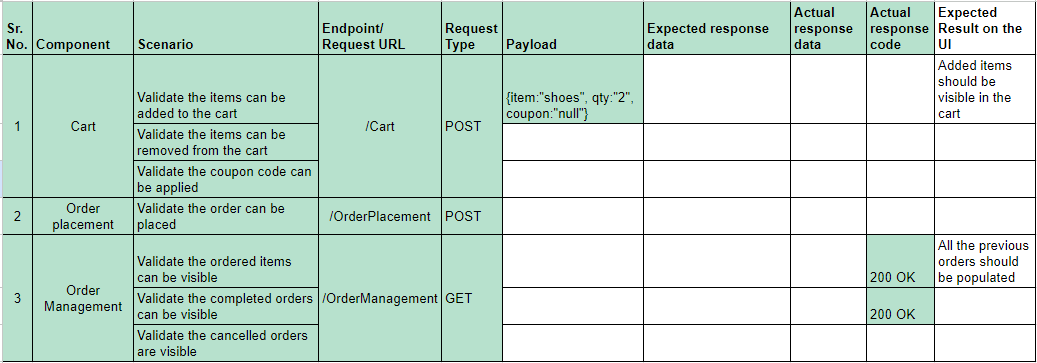
Integration testing: As we know that microservices communicate with each other through APIs. Thus, API testing plays an important role in this phase. In this testing phase, we need to validate the interfacing and integration aspects of the microservices. The goal of integration testing is to ensure the data flow from one component to another, in other words, validate how the components work together. Prepare the test scenarios that cover the interaction points of the components.
For e.g. Adding to cart --> Placing the order --> Checking the previous orders
Here, you are validating the request and response of components working together.
The following tools can be used for API testing:
1. Postman
2. Java RestAssured API
3. SoupUI
Contract testing: Contract testing is an enhanced form of integration testing. It is a methodology that captures the communication between two independent systems (microservices). The captured interaction is stored in a contract and later the validation is performed to check whether the systems are adhering to this contract or not.
End to end testing: E2E testing should cover the entire logical flow of the application from start to end. E2E testing is built on integration testing and it should cover the complete functional paths of the application from one start to end.
How to write test cases for testing microservices
Understand, what each microservice is supposed to do and figure out the endpoints. Many a time, the dev team prepare
the detailed doc that contains the endpoints, request URLs, their functionalities, required payload, headers details, request types, etc.
But in the absence of that, we need to take out these details by manually performing the UI operations on the browser and fetching the
details through the browser's network tab.
The key performance indicators from this doc should be the response data that came out after executing a specified set of scenarios.
List down the scenarios, and identify the request URL, header details, payload (test data), expected response, and actual response. Once all these details are identified, prepare a test case doc with the specified headers as shown below:
Executing the API tests through Java rest-assured
1. First things first.
Add all the required rest-assured dependencies in pom.xml
2. You need to perform the authentication before performing the API tests.
You can perform the cookie-based authentication in case your app is using cookies for token id or auth id. For this write a UI test using Selenium and Java and perform the login operation.
Now once the login is done, you can fetch the cookies using Selenium's getCookies() function, store them in a set, and return the set of cookies in your API tests :
3. In the API test, define the base URI:
4. Create request and response objects, pass the cookies in the headers:
req.header("Content-Type","application/json");
Iterator r=s.iterator();
while(r.hasNext()) {
try {
req.header("cookie",r.next().toString());
}
catch(Exception e) {
test.log(LogStatus.FAIL, "Failed to pass the cookies to the request headers" +e.getMessage());
}
}
6. Send the request using the request object, and store the response in the response object.
7. Analyze the response :
res.getBody().asString()
res.asPrettyString()
8. Also, fetch the required data from the response that can be passed in the subsequent request: